Google Authentication React Component
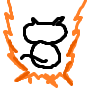
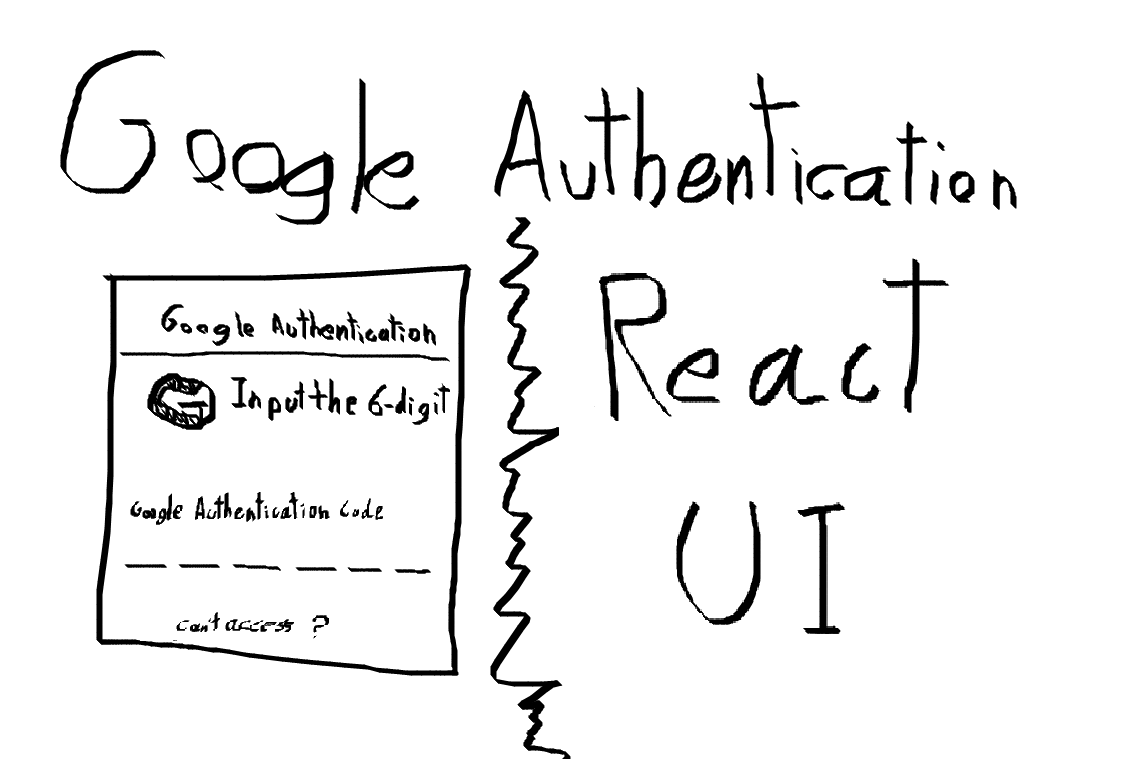
ช่องอินพุตทั้งหมดมี 6 ช่องอินพุตเก็บเก็บค่าเป็น string ขนาดความยาวไม่เกิน 6 ตัวอักษรหลังจากกรอกครบสามารถเขียนโค้ดต่อได้ว่าจะให้ทำอะไร
การทำงานของโปรแกรมที่ต้องการ
- เก็บข้อมูลเป็น string 6 ตัวอักษร
- 1 อินพุตต่อ 1 ตัวอักษร
let input = "123456";<input value={input[0]} /><input value={input[1]} /><input value={input[2]} /><input value={input[3]} /><input value={input[4]} /><input value={input[5]} />
- หลังจากอินพุตแล้ว focus อินพุตถัดไป
- เมื่อลบก็ต้องลบแล้ว focus อินพุตช่องสุดท้ายที่มีข้อมูลอยู่
- สามารถ paste from clipboard (CTRL+V) ได้
เครื่องมือที่ใช้งาน
- react
- css modules
import React, { useEffect, useState } from "react";import "./App.css";
import styles from "./twofa.module.css";
function App() { const initialSetting = { max: 6, onChangeValue: () => {}, }; const [setting, setSetting] = useState(initialSetting);
const [input, setInput] = useState("");
const handleChange = (e) => { try { if (e.target.value) { let { value } = e.target;
if (input.length > 0) { // when the data already exists if (input.length < setting.max) { if (input[e.target.attributes.tabindex.value - 1]?.length > 0) { value = value.substring(1); }
let data = input.concat(value); if (data.length > 6) { data = data.slice(0, 6); } setInput(data);
document.getElementById(`pin${data.length}`).focus(); } } else { // when empty data if (value.length > 6) { value = value.slice(0, 6); } setInput(value); document.getElementById(`pin${value.length}`).focus(); } } else { // clear data if (input.length > 0) { setInput(input.slice(0, -1));
if (input.length !== 1) { document.getElementById(`pin${input.length - 1}`).focus(); } } } } catch (error) { console.log("some error"); } };
useEffect(() => { console.log(input); if (input.length === 6) { // code... console.log("Do Something..."); } }, [input]); return ( <React.Fragment> <div className={styles.wrapper__size}> <div className={styles.twofa_blur}></div> <div className={styles.twofa}> <div className={styles.twofa__header}> <div className={styles.twofa__header__title}> <span>Google Authentication</span> </div> </div> <div className={styles.twofa__body}> <div className={styles.twofa__body__flex}> <div className={styles.twofa__body__flex__flex}> <div className={styles.twofa__body__flex__flex__message}> <div className={styles.twofa__body__flex__flex__message__flex} > <div className={ styles.twofa__body__flex__flex__message__flex__img } > <img width="60" src="images/Google_Authenticator_for_Android_icon.png" /> </div> <div className={ styles.twofa__body__flex__flex__message__flex__text } > <span> Input the 6-digit code in your Google Authenticator app </span> </div> </div> </div> <div className={styles.twofa__body__flex__flex__input}> <div className={styles.twofa__body__flex__flex__input__flex}> <div className={ styles.twofa__body__flex__flex__input__flex__message } > <span>Google Authentication Code</span> </div> <div className={ styles.twofa__body__flex__flex__input__flex__input } > <input id="pin1" // maxLength="1" tabIndex="1" value={input[0] ? input[0] : ""} onChange={handleChange} autoFocus={true} /> <input id="pin2" // maxLength="1" tabIndex="2" value={input[1] ? input[1] : ""} onChange={handleChange} /> <input id="pin3" // maxLength="1" tabIndex="3" value={input[2] ? input[2] : ""} onChange={handleChange} /> <input id="pin4" // maxLength="1" tabIndex="4" value={input[3] ? input[3] : ""} onChange={handleChange} /> <input id="pin5" // maxLength="1" tabIndex="5" value={input[4] ? input[4] : ""} onChange={handleChange} /> <input id="pin6" // maxLength="1" tabIndex="6" value={input[5] ? input[5] : ""} onChange={handleChange} /> </div> </div> </div> </div> <div className={styles.twofa__body__flex__help}> <span>Can't access Google Authenticator?</span> </div> </div> </div> {/* <div className={styles.twofa__footer}></div> */} </div> </div> </React.Fragment> );}
export default App;
* { margin: 0; padding: 0; box-sizing: border-box;}* { font-size: 16px;}.noselect { -webkit-touch-callout: none; /* iOS Safari */ -webkit-user-select: none; /* Safari */ -khtml-user-select: none; /* Konqueror HTML */ -moz-user-select: none; /* Old versions of Firefox */ -ms-user-select: none; /* Internet Explorer/Edge */ user-select: none; /* Non-prefixed version, currently supported by Chrome, Edge, Opera and Firefox */}.wrapper__size { min-height: 100vh; display: block; position: absolute; left: 0; right: 0; top: 0;}.twofa { position: absolute; margin-left: auto; margin-right: auto; left: 0; right: 0; text-align: center; width: 100%; max-width: 500px; margin-top: 50px; z-index: 9999; background-color: rgba(255, 255, 255, 1); box-shadow: rgb(0 0 0 / 8%) 0px 6px 10px;}.twofa_blur { filter: blur(8px); -webkit-filter: blur(8px); /* background-image: url(https://source.unsplash.com/random); */ background-color: rgba(200, 200, 200, 1); width: 100%; height: 100vh; position: absolute;}.twofa__header {}.twofa__header__title { padding: 20px 40px;}.twofa__header__title span { font-size: 1.2em; font-weight: 700;}.twofa__body {}.twofa__body__flex { display: flex; flex-direction: column;
justify-content: space-between; min-height: 350px;}.twofa__body__flex__flex { display: flex; flex-direction: column;}.twofa__body__flex__flex__message { padding: 20px 40px;}.twofa__body__flex__flex__message__flex { display: flex; flex-direction: row; /* justify-content: flex-start; */ align-items: center; text-align: left;}.twofa__body__flex__flex__message__flex__img {}.twofa__body__flex__flex__message__flex__img img { margin-right: 10px;}.twofa__body__flex__flex__message__flex__text {}.twofa__body__flex__flex__input { padding: 20px 40px;}.twofa__body__flex__flex__input__flex { text-align: left;}.twofa__body__flex__flex__input__flex__message {}.twofa__body__flex__flex__input__flex__message span { color: rgba(125, 125, 125, 1);}.twofa__body__flex__flex__input__flex__input { display: grid; grid-template-columns: auto auto auto auto auto auto;}.twofa__body__flex__flex__input__flex__input input { max-width: 50px; min-height: 50px; /* padding: 10px; */
font-size: 1.2em; font-weight: 500; text-align: center;
border-left: none; border-right: none; border-top: none; border-bottom: 2px solid rgba(200, 200, 200, 1);}.twofa__body__flex__flex__input__flex__input input:focus { outline: none;}.twofa__body__flex__help { padding: 20px;}.twofa__body__flex__help span { color: rgba(241, 191, 29, 1); font-weight: 700;}
สุดท้าย ก็หวังว่าบทความนี้จะเป็นประโยชน์กับใครหลาย ๆ คนนะครับ